Working with missing data¶
In this section, we will discuss missing (also referred to as NA) values in pandas.
Note
The choice of using NaN internally to denote missing data was largely for simplicity and performance reasons. It differs from the MaskedArray approach of, for example, scikits.timeseries. We are hopeful that NumPy will soon be able to provide a native NA type solution (similar to R) performant enough to be used in pandas.
Missing data basics¶
When / why does data become missing?¶
Some might quibble over our usage of missing. By “missing” we simply mean null or “not present for whatever reason”. Many data sets simply arrive with missing data, either because it exists and was not collected or it never existed. For example, in a collection of financial time series, some of the time series might start on different dates. Thus, values prior to the start date would generally be marked as missing.
In pandas, one of the most common ways that missing data is introduced into a data set is by reindexing. For example
In [732]: df = DataFrame(randn(5, 3), index=['a', 'c', 'e', 'f', 'h'],
.....: columns=['one', 'two', 'three'])
In [733]: df['four'] = 'bar'
In [734]: df['five'] = df['one'] > 0
In [735]: df
Out[735]:
one two three four five
a 0.059117 1.138469 -2.400634 bar True
c -0.280853 0.025653 -1.386071 bar False
e 0.863937 0.252462 1.500571 bar True
f 1.053202 -2.338595 -0.374279 bar True
h -2.359958 -1.157886 -0.551865 bar False
In [736]: df2 = df.reindex(['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h'])
In [737]: df2
Out[737]:
one two three four five
a 0.059117 1.138469 -2.400634 bar True
b NaN NaN NaN NaN NaN
c -0.280853 0.025653 -1.386071 bar False
d NaN NaN NaN NaN NaN
e 0.863937 0.252462 1.500571 bar True
f 1.053202 -2.338595 -0.374279 bar True
g NaN NaN NaN NaN NaN
h -2.359958 -1.157886 -0.551865 bar False
Values considered “missing”¶
As data comes in many shapes and forms, pandas aims to be flexible with regard to handling missing data. While NaN is the default missing value marker for reasons of computational speed and convenience, we need to be able to easily detect this value with data of different types: floating point, integer, boolean, and general object. In many cases, however, the Python None will arise and we wish to also consider that “missing” or “null”. Lastly, for legacy reasons inf and -inf are also considered to be “null” in computations. Since in NumPy divide-by-zero generates inf or -inf and not NaN, I think you will find this is a worthwhile trade-off (Zen of Python: “practicality beats purity”).
To make detecting missing values easier (and across different array dtypes), pandas provides the isnull() and notnull() functions, which are also methods on Series objects:
In [738]: df2['one']
Out[738]:
a 0.059117
b NaN
c -0.280853
d NaN
e 0.863937
f 1.053202
g NaN
h -2.359958
Name: one
In [739]: isnull(df2['one'])
Out[739]:
a False
b True
c False
d True
e False
f False
g True
h False
Name: one
In [740]: df2['four'].notnull()
Out[740]:
a True
b False
c True
d False
e True
f True
g False
h True
Summary: NaN, inf, -inf, and None (in object arrays) are all considered missing by the isnull and notnull functions.
Calculations with missing data¶
Missing values propagate naturally through arithmetic operations between pandas objects.
In [741]: a
Out[741]:
one two
a 0.059117 1.138469
b 0.059117 1.138469
c -0.280853 0.025653
d -0.280853 0.025653
e 0.863937 0.252462
In [742]: b
Out[742]:
one two three
a 0.059117 1.138469 -2.400634
b NaN NaN NaN
c -0.280853 0.025653 -1.386071
d NaN NaN NaN
e 0.863937 0.252462 1.500571
In [743]: a + b
Out[743]:
one three two
a 0.118234 NaN 2.276938
b NaN NaN NaN
c -0.561707 NaN 0.051306
d NaN NaN NaN
e 1.727874 NaN 0.504923
The descriptive statistics and computational methods discussed in the data structure overview (and listed here and here) are all written to account for missing data. For example:
- When summing data, NA (missing) values will be treated as zero
- If the data are all NA, the result will be NA
- Methods like cumsum and cumprod ignore NA values, but preserve them in the resulting arrays
In [744]: df
Out[744]:
one two three
a 0.059117 1.138469 -2.400634
b NaN NaN NaN
c -0.280853 0.025653 -1.386071
d NaN NaN NaN
e 0.863937 0.252462 1.500571
f 1.053202 -2.338595 -0.374279
g NaN NaN NaN
h -2.359958 -1.157886 -0.551865
In [745]: df['one'].sum()
Out[745]: -0.66455558290247652
In [746]: df.mean(1)
Out[746]:
a -0.401016
b NaN
c -0.547090
d NaN
e 0.872323
f -0.553224
g NaN
h -1.356570
In [747]: df.cumsum()
Out[747]:
one two three
a 0.059117 1.138469 -2.400634
b NaN NaN NaN
c -0.221736 1.164122 -3.786705
d NaN NaN NaN
e 0.642200 1.416584 -2.286134
f 1.695403 -0.922011 -2.660413
g NaN NaN NaN
h -0.664556 -2.079897 -3.212278
NA values in GroupBy¶
NA groups in GroupBy are automatically excluded. This behavior is consistent with R, for example.
Cleaning / filling missing data¶
pandas objects are equipped with various data manipulation methods for dealing with missing data.
Filling missing values: fillna¶
The fillna function can “fill in” NA values with non-null data in a couple of ways, which we illustrate:
Replace NA with a scalar value
In [748]: df2
Out[748]:
one two three four five
a 0.059117 1.138469 -2.400634 bar True
b NaN NaN NaN NaN NaN
c -0.280853 0.025653 -1.386071 bar False
d NaN NaN NaN NaN NaN
e 0.863937 0.252462 1.500571 bar True
f 1.053202 -2.338595 -0.374279 bar True
g NaN NaN NaN NaN NaN
h -2.359958 -1.157886 -0.551865 bar False
In [749]: df2.fillna(0)
Out[749]:
one two three four five
a 0.059117 1.138469 -2.400634 bar True
b 0.000000 0.000000 0.000000 0 0
c -0.280853 0.025653 -1.386071 bar False
d 0.000000 0.000000 0.000000 0 0
e 0.863937 0.252462 1.500571 bar True
f 1.053202 -2.338595 -0.374279 bar True
g 0.000000 0.000000 0.000000 0 0
h -2.359958 -1.157886 -0.551865 bar False
In [750]: df2['four'].fillna('missing')
Out[750]:
a bar
b missing
c bar
d missing
e bar
f bar
g missing
h bar
Name: four
Fill gaps forward or backward
Using the same filling arguments as reindexing, we can propagate non-null values forward or backward:
In [751]: df
Out[751]:
one two three
a 0.059117 1.138469 -2.400634
b NaN NaN NaN
c -0.280853 0.025653 -1.386071
d NaN NaN NaN
e 0.863937 0.252462 1.500571
f 1.053202 -2.338595 -0.374279
g NaN NaN NaN
h -2.359958 -1.157886 -0.551865
In [752]: df.fillna(method='pad')
Out[752]:
one two three
a 0.059117 1.138469 -2.400634
b 0.059117 1.138469 -2.400634
c -0.280853 0.025653 -1.386071
d -0.280853 0.025653 -1.386071
e 0.863937 0.252462 1.500571
f 1.053202 -2.338595 -0.374279
g 1.053202 -2.338595 -0.374279
h -2.359958 -1.157886 -0.551865
To remind you, these are the available filling methods:
Method | Action |
---|---|
pad / ffill | Fill values forward |
bfill / backfill | Fill values backward |
With time series data, using pad/ffill is extremely common so that the “last known value” is available at every time point.
Dropping axis labels with missing data: dropna¶
You may wish to simply exclude labels from a data set which refer to missing data. To do this, use the dropna method:
In [753]: df
Out[753]:
one two three
a 0.059117 1.138469 -2.400634
b NaN 0.000000 0.000000
c -0.280853 0.025653 -1.386071
d NaN 0.000000 0.000000
e 0.863937 0.252462 1.500571
f 1.053202 -2.338595 -0.374279
g NaN 0.000000 0.000000
h -2.359958 -1.157886 -0.551865
In [754]: df.dropna(axis=0)
Out[754]:
one two three
a 0.059117 1.138469 -2.400634
c -0.280853 0.025653 -1.386071
e 0.863937 0.252462 1.500571
f 1.053202 -2.338595 -0.374279
h -2.359958 -1.157886 -0.551865
In [755]: df.dropna(axis=1)
Out[755]:
two three
a 1.138469 -2.400634
b 0.000000 0.000000
c 0.025653 -1.386071
d 0.000000 0.000000
e 0.252462 1.500571
f -2.338595 -0.374279
g 0.000000 0.000000
h -1.157886 -0.551865
In [756]: df['one'].dropna()
Out[756]:
a 0.059117
c -0.280853
e 0.863937
f 1.053202
h -2.359958
Name: one
dropna is presently only implemented for Series and DataFrame, but will be eventually added to Panel. Series.dropna is a simpler method as it only has one axis to consider. DataFrame.dropna has considerably more options, which can be examined in the API.
Interpolation¶
A basic linear interpolate method has been implemented on Series with intended use for time series data. There has not been a great deal of demand for interpolation methods outside of the filling methods described above.
In [757]: fig, axes = plt.subplots(ncols=2, figsize=(8, 4))
In [758]: ts.plot(ax=axes[0])
Out[758]: <matplotlib.axes.AxesSubplot at 0x1124b1410>
In [759]: ts.interpolate().plot(ax=axes[1])
Out[759]: <matplotlib.axes.AxesSubplot at 0x10e803bd0>
In [760]: axes[0].set_title('Not interpolated')
Out[760]: <matplotlib.text.Text at 0x1133b0f90>
In [761]: axes[1].set_title('Interpolated')
Out[761]: <matplotlib.text.Text at 0x1146c0790>
In [762]: plt.close('all')
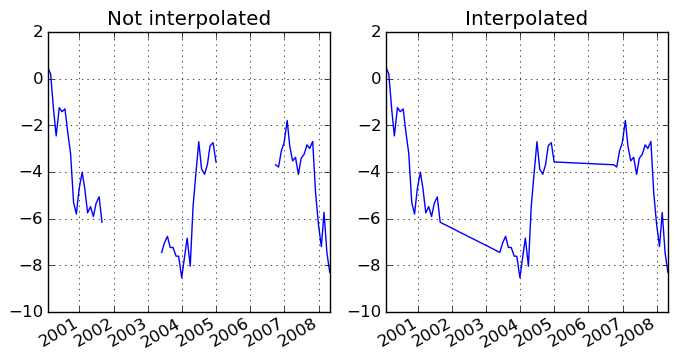
Missing data casting rules and indexing¶
While pandas supports storing arrays of integer and boolean type, these types are not capable of storing missing data. Until we can switch to using a native NA type in NumPy, we’ve established some “casting rules” when reindexing will cause missing data to be introduced into, say, a Series or DataFrame. Here they are:
data type | Cast to |
---|---|
integer | float |
boolean | object |
float | no cast |
object | no cast |
For example:
In [763]: s = Series(randn(5), index=[0, 2, 4, 6, 7])
In [764]: s > 0
Out[764]:
0 False
2 True
4 True
6 True
7 True
In [765]: (s > 0).dtype
Out[765]: dtype('bool')
In [766]: crit = (s > 0).reindex(range(8))
In [767]: crit
Out[767]:
0 False
1 NaN
2 True
3 NaN
4 True
5 NaN
6 True
7 True
In [768]: crit.dtype
Out[768]: dtype('object')
Ordinarily NumPy will complain if you try to use an object array (even if it contains boolean values) instead of a boolean array to get or set values from an ndarray (e.g. selecting values based on some criteria). If a boolean vector contains NAs, an exception will be generated:
In [769]: reindexed = s.reindex(range(8)).fillna(0)
In [770]: reindexed[crit]
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-770-2da204ed1ac7> in <module>()
----> 1 reindexed[crit]
/Users/changshe/code/pandas/pandas/core/series.py in __getitem__(self, key)
392 # special handling of boolean data with NAs stored in object
393 # arrays. Since we can't represent NA with dtype=bool
--> 394 if _is_bool_indexer(key):
395 key = self._check_bool_indexer(key)
396 key = np.asarray(key, dtype=bool)
/Users/changshe/code/pandas/pandas/core/common.py in _is_bool_indexer(key)
330 if not lib.is_bool_array(key):
331 if isnull(key).any():
--> 332 raise ValueError('cannot index with vector containing '
333 'NA / NaN values')
334 return False
ValueError: cannot index with vector containing NA / NaN values
However, these can be filled in using fillna and it will work fine:
In [771]: reindexed[crit.fillna(False)]
Out[771]:
2 1.314232
4 0.690579
6 0.995761
7 2.396780
In [772]: reindexed[crit.fillna(True)]
Out[772]:
1 0.000000
2 1.314232
3 0.000000
4 0.690579
5 0.000000
6 0.995761
7 2.396780